Using Reference Aliases
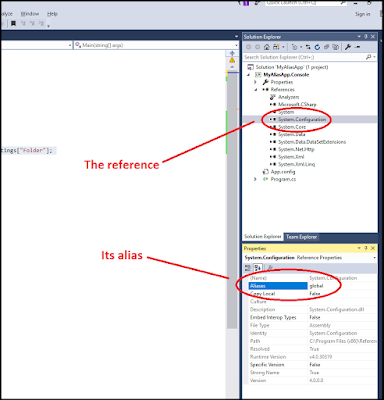
I recently ran across a situation where two different libraries shared identical namespaces and class names. Visual Studio complained anytime I used one of these classes that it was an ambiguous reference. I needed both libraries -- each contained some unique elements -- so I couldn't ditch one or the other. But, as I said, anytime I tried to use a class that was common (again, via the class name and namespace), I received the ambiguous reference error. This was a real problem, so say the least. However, C# provides a way to specify exactly which library (reference) you mean when you use an object that's common to two or more libraries. Simply put, you use an extern alias statement. The concept is fairly simple: Give the reference an alias (I used the Properties windows for the reference). Add an extern alias statement, putting it before your using statements. Add a using statement, which effectively links a specific object through the extern alias statement to th...